Mastering Python: A Comprehensive Guide to Modules and Packages
Written on
Chapter 1: Introduction to Python's Code Structure
In the expansive realm of Python programming, the significance of organizing code and ensuring its reusability cannot be overstated. This is where modules and packages come into play, serving as essential tools that enhance your code's clarity, modularity, and maintainability. This article will explore the fundamentals of modules and packages, providing practical examples to help you structure your Python projects more effectively.
Understanding Modules
What Exactly are Modules?
At its essence, a module in Python refers to a file that houses Python definitions, functions, and statements. It serves as a method to organize your code, making it easier to read and maintain.
Creating and Importing Modules
Let’s look at a straightforward example. Suppose you have a file named math_operations.py containing these functions:
# math_operations.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
You can then import and utilize these functions in another Python script as follows:
# main.py
import math_operations
result_addition = math_operations.add(5, 3)
result_subtraction = math_operations.subtract(10, 4)
print(f"Addition Result: {result_addition}")
print(f"Subtraction Result: {result_subtraction}")
This modular approach consolidates related functionalities, helping to reduce clutter in your main script.
Embracing Packages
What are Packages?
As your project expands, a single module may not suffice. This is where packages come in, which are collections of modules organized within a directory. A package must contain an __init__.py file (which can be empty) to indicate that the directory should be recognized as a package.
Creating and Using Packages
Consider a package named shapes that includes modules like circle.py and rectangle.py:
# shapes/circle.py
import math
def area(radius):
return math.pi * radius ** 2
# shapes/rectangle.py
def area(length, width):
return length * width
You can import and apply these modules in your main script like this:
# main.py
from shapes import circle, rectangle
circle_area = circle.area(5)
rectangle_area = rectangle.area(3, 4)
print(f"Circle Area: {circle_area}")
print(f"Rectangle Area: {rectangle_area}")
This structured organization allows you to keep related functionalities grouped, making your project layout more intuitive.
Practical Examples: Real-world Applications
Example 1: Utilizing a Utility Module
Let’s examine a utility module named helper_functions.py:
# helper_functions.py
def square(number):
return number ** 2
def double(number):
return number * 2
You can use this utility module across different scripts:
# main1.py
from helper_functions import square
result = square(4)
print(f"Square Result: {result}")
# main2.py
from helper_functions import double
result = double(7)
print(f"Double Result: {result}")
This modular method enables the reuse of common functionalities throughout various parts of your project.
Example 2: Creating a Package for Data Processing
Imagine you are engaged in a data processing project with modules for managing CSV and JSON data:
# data_processing/csv_handler.py
import csv
def read_csv(file_path):
# Implementation for reading CSV files
pass
# data_processing/json_handler.py
import json
def read_json(file_path):
# Implementation for reading JSON files
pass
Your main script can take advantage of these modules:
# main_data_processing.py
from data_processing import csv_handler, json_handler
csv_data = csv_handler.read_csv("data.csv")
json_data = json_handler.read_json("data.json")
# Further processing of data...
This modular design allows you to adjust or enhance specific components without impacting the entire project.
Best Practices: Tips for Effective Module and Package Usage
Keep it Modular
Break your code down into concise, logical modules. Each module should manage a specific set of functionalities, promoting better readability and maintainability.
Organize with Packages
As your project grows, think about organizing related modules into packages. This hierarchical structure simplifies navigation and maintenance.
Use Descriptive Names
Select clear and succinct names for your modules and packages. This practice enhances code readability and aids others in understanding the purpose of each component.
Conclusion: Structuring Python Projects Like a Pro
Modules and packages in Python are invaluable tools for organizing code, fostering reusability, and maintaining a clean project structure. With this foundational knowledge, you can confidently structure your Python projects for efficiency and scalability.
Engage in your coding journeys, decompose complex tasks into modular components, and harness the power of Python's building blocks. Regardless of whether you are a novice or a seasoned developer, mastering modules and packages will significantly enhance your coding experience.
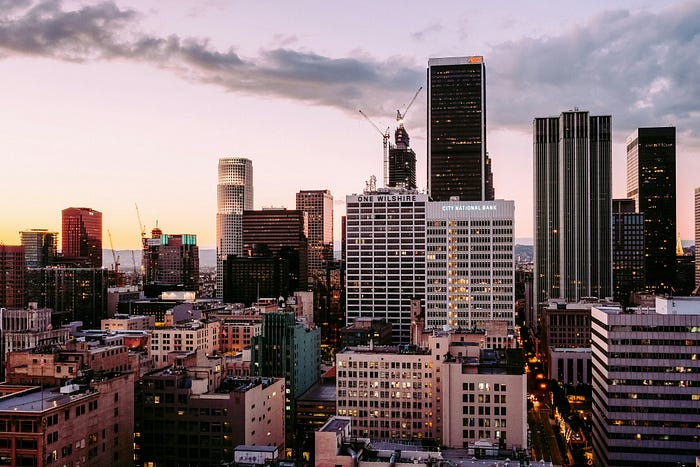
Explore the fundamentals of modules and packages in Python programming, perfect for beginners looking to organize their code effectively.
Join this tutorial designed for beginners to understand modules and packages in Python, featuring hands-on examples and explanations.