Mastering Array Manipulation: Remove the Last Element in JavaScript
Written on
Chapter 1: Introduction to Array Manipulation
In programming, particularly with JavaScript, it is often necessary to remove the last element from an array. This article explores several methods to achieve this.
When working with arrays, the ability to modify them dynamically is crucial, especially when it comes to removing elements.
Section 1.1: Using Array.prototype.splice
One effective method to remove the last element from a JavaScript array is by utilizing the splice method. For example:
const array = [1, 2, 3];
array.splice(-1, 1);
console.log(array);
In this code, we invoke splice with -1 to target the last element, while the second argument signifies the number of items to remove. Consequently, the array is modified to [1, 2].
Subsection 1.1.1: Visual Explanation
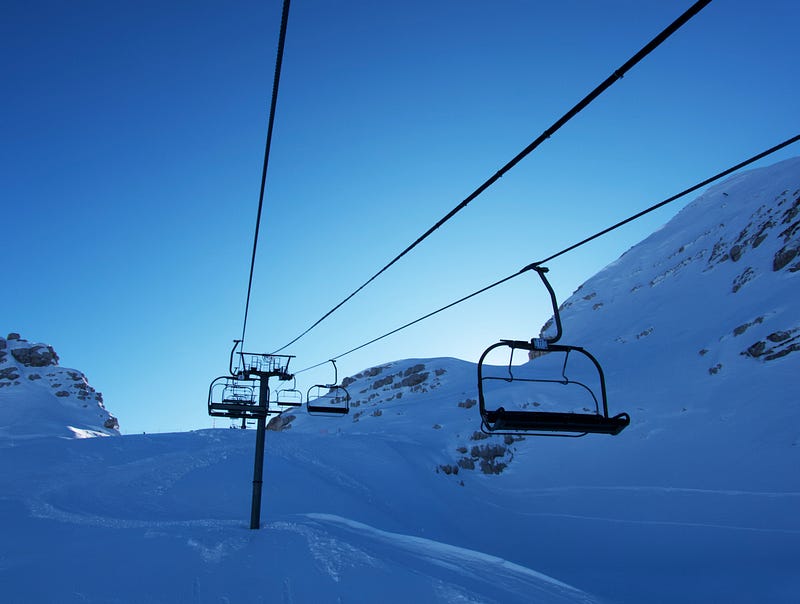
Section 1.2: Using Array.prototype.pop
Another straightforward method is the pop function. This method not only removes the last element but also returns it. For instance:
const array = [1, 2, 3];
const popped = array.pop();
console.log(popped, array);
Here, calling pop removes the final element from the array and assigns it to the variable popped, which would yield 3, leaving the array as [1, 2].
Chapter 2: Other Methods for Removing Elements
In addition to splice and pop, there are other methods available for removing the last item from an array.
The first video titled "How to remove the last element of a JavaScript Array - Array.prototype.pop()" provides a clear demonstration of how the pop method works in practice.
The second video "how to remove the last element from an array in javascript" further elaborates on techniques for manipulating arrays, including practical examples.
Section 2.1: Using Array.prototype.slice
Another method for removing the last element is through the slice function. This function allows you to create a new array by specifying start and end indices. For example:
const array = [1, 2, 3];
const newArr = array.slice(0, -1);
console.log(newArr);
In this case, calling slice with 0 as the start index and -1 as the end index results in a new array that excludes the last element, yielding [1, 2].
Section 2.2: Utilizing Array.prototype.filter
Additionally, the filter method can be employed to create an array that excludes the last element based on specific conditions. Here’s how you can implement it:
const array = [1, 2, 3];
const newArr = array.filter((element, index) => index < array.length - 1);
console.log(newArr);
In this example, we pass a callback function to filter, which ensures that only elements with an index less than the last index are retained, thereby generating the same result of [1, 2].
Conclusion
In summary, there are multiple JavaScript array methods available for removing the last item from an array, each with its own unique approach. Understanding these methods can enhance your coding proficiency and array manipulation skills.