Removing Objects from a JavaScript Array Using Object Properties
Written on
Chapter 1: Understanding Object Removal
In JavaScript, it is sometimes necessary to eliminate an object from an array based on a certain property value. This tutorial will guide you through the steps to achieve this.
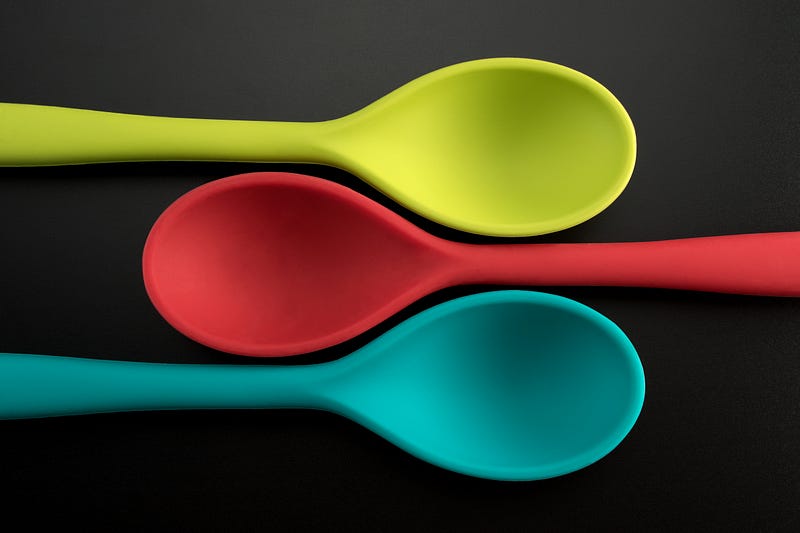
Section 1.1: Utilizing Array Methods
We can leverage the Array.prototype.findIndex method to locate the index of the object within the array that possesses the specified property value. After identifying the index, we can employ the Array.prototype.splice method to remove the object at that index.
For example, consider the following array:
const items = [{
id: 'abc',
name: 'oh'
},
{
id: 'efg',
name: 'em'
},
{
id: 'hij',
name: 'ge'
}
];
To find and remove the object with the id of 'abc', we can implement the following code:
const index = items.findIndex((i) => {
return i.id === "abc";
});
items.splice(index, 1);
console.log(items);
After executing this code, the items array will be modified to:
[
{
"id": "efg",
"name": "em"
},
{
"id": "hij",
"name": "ge"
}
]
Section 1.2: Conclusion
By using the findIndex method, we can efficiently identify the index of an object that meets a specific condition within an array. Subsequently, the splice method allows us to remove that object based on the index obtained from findIndex.
Chapter 2: Video Tutorials
To further enhance your understanding, here are some helpful video resources:
This video titled "How to remove array element based on object property in Javascript?" provides a practical demonstration of the concepts discussed.
Another useful video, "JavaScript's Delete Operator - How to Delete Object Properties with One Command," elaborates on deleting object properties effectively.