Finding the Size of Local Storage Using JavaScript Techniques
Written on
Chapter 1 Understanding Local Storage Size
In web development, knowing the size of your browser's local storage can be essential. This guide will explore methods to ascertain the size of local storage using JavaScript.
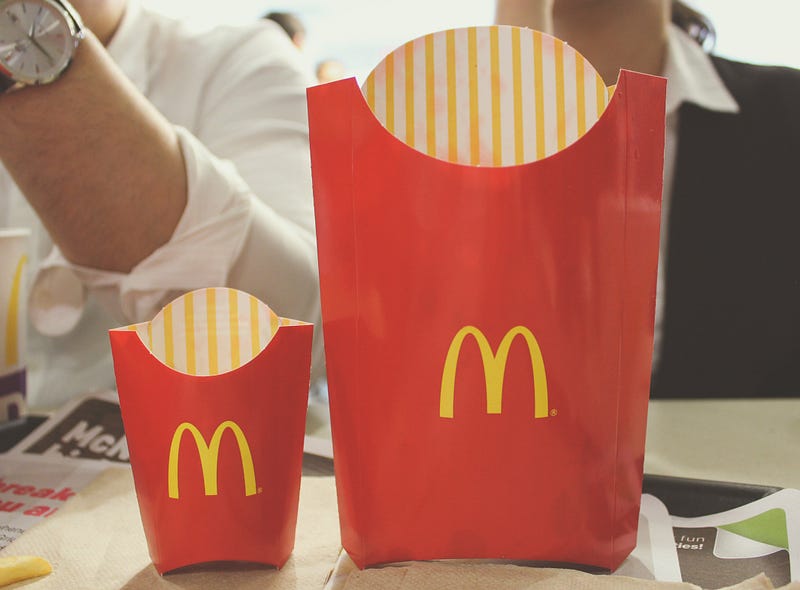
Section 1.1 Converting Local Storage to Blob
To determine the size of the local storage object, one effective approach is to convert it into a Blob. This process allows us to access the size property.
The code snippet below demonstrates this method:
const { size } = new Blob(Object.values(localStorage));
console.log(size);
Here, we utilize Object.values to retrieve an array of values from local storage, which we then pass to the Blob constructor. This enables us to access the size of the blob, effectively giving us the size of our local storage.
Section 1.2 Calculating Size in Kilobytes
To express the size in kilobytes, we can create a custom function that calculates the total number of bytes stored in local storage and converts that figure into kilobytes.
Here’s an example function:
const localStorageSpace = () => {
let allStrings = '';
for (const key of Object.keys(window.localStorage)) {
allStrings += window.localStorage[key];}
return allStrings ? 3 + ((allStrings.length * 16) / (8 * 1024)) + ' KB' : 'Empty (0 KB)';
};
console.log(localStorageSpace());
In this function, we concatenate all stored strings into the variable allStrings. The size is computed by taking the length of this string, multiplying it by 16, and then dividing by (8 times 1024) to convert the result into kilobytes. We also add 3 to account for overhead.
Chapter 2 Conclusion
By converting local storage into a blob, we can easily determine its size. Additionally, crafting a custom function allows us to express that size in kilobytes for a clearer understanding of our storage use.
For further insights, you can explore more content at PlainEnglish.io. Consider subscribing to our free weekly newsletter, and connect with us on Twitter, LinkedIn, YouTube, and Discord.
The first video explains how to check localStorage space in JavaScript, providing a practical tutorial on the topic.
The second video covers the use of local storage in JavaScript, offering a comprehensive guide to understanding and implementing this feature.