Mastering Hapi.js: Effective 404 Handling and Method Sharing
Written on
Chapter 1: Introduction to Hapi.js
Hapi.js is a lightweight Node.js framework designed for back-end web application development. In this article, we will explore how to effectively create back-end applications using Hapi.js.
Section 1.1: Handling 404 Errors
Managing 404 errors in Hapi is straightforward. For instance, you can implement a route that captures all paths that do not match existing routes. Here's an example:
const Hapi = require('@hapi/hapi');
const init = async () => {
const server = Hapi.server({
port: 3000,
host: '0.0.0.0'
});
server.route({
method: '*',
path: '/{any*}',
handler(request, h) {
return '404 Error! Page Not Found!';}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
In this code, the method is set to '*' to capture all HTTP methods, while the path /{any*} enables the route handler to monitor any URL path.
Subsection 1.1.1: Visual Guide to Hapi Validation
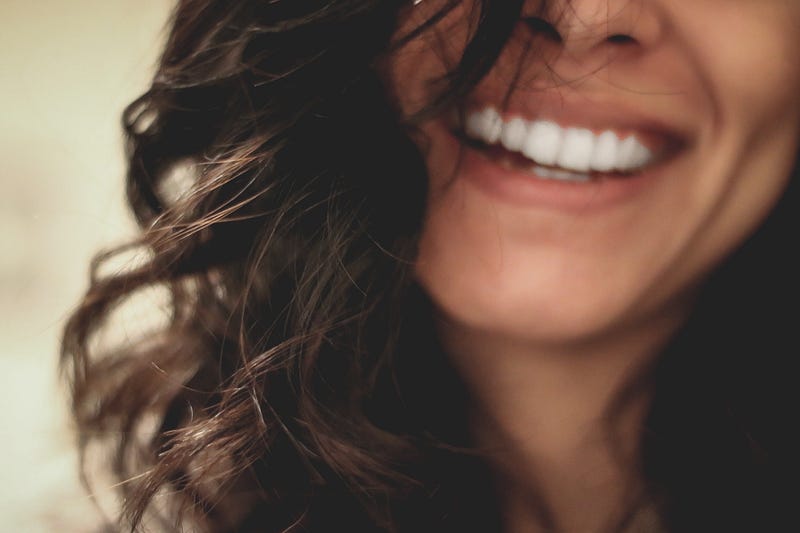
Section 1.2: Utilizing Server Methods
The server.method function allows you to add reusable methods throughout your application. For example, consider the following implementation:
const Hapi = require('@hapi/hapi');
const add = (x, y) => {
return x + y;
};
const init = async () => {
const server = Hapi.server({
port: 3000,
host: '0.0.0.0'
});
server.method('add', add, {});
server.route({
method: 'GET',
path: '/',
handler(request, h) {
return server.methods.add(1, 2);}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
Here, we define an add function that sums two numbers. We then register this function as a method on the server, making it accessible throughout the application. When we visit the root path, it returns the result of adding 1 and 2, yielding a response of 3.
Chapter 2: Advanced Method Registration
You can also register methods using an object format, which can be particularly useful for organizing your code:
const Hapi = require('@hapi/hapi');
const add = (x, y) => {
return x + y;
};
const init = async () => {
const server = Hapi.server({
port: 3000,
host: '0.0.0.0'
});
server.method({
name: 'add',
method: add,
options: {}
});
server.route({
method: 'GET',
path: '/',
handler(request, h) {
return server.methods.add(1, 2);}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
This approach achieves the same functionality as the previous example but uses an object to define the method registration.
The first video, "Hapi Validation and Error Handling," provides an insightful overview of managing validation and error handling within Hapi applications.
You can also namespace your methods to organize them further. For instance:
const Hapi = require('@hapi/hapi');
const add = (x, y) => {
return x + y;
};
const init = async () => {
const server = Hapi.server({
port: 3000,
host: '0.0.0.0'
});
server.method('math.add', add);
server.route({
method: 'GET',
path: '/',
handler(request, h) {
return server.methods.math.add(1, 2);}
});
await server.start();
console.log('Server running on %s', server.info.uri);
};
process.on('unhandledRejection', (err) => {
console.log(err);
process.exit(1);
});
init();
In this example, we register the add method under the namespace math, allowing for better organization and categorization of methods.
The second video, "Global Error Handling Middlewares on a TypeScript Express React Project," discusses how to implement error handling strategies across different frameworks and languages.
Conclusion
In summary, Hapi.js simplifies the process of managing 404 errors and allows for the creation of shared methods across your application, making it a powerful framework for back-end development.