Mastering JavaScript Fundamentals: A 100-Day Journey
Written on
Day 1: An Introduction to JavaScript Basics
On March 23, 2024, we kick off our journey into JavaScript by exploring crucial topics including variables, data types, operators, control flow, and other engaging subjects each day. This comprehensive guide aims to prepare you thoroughly for your upcoming JavaScript interview.
We will document a 100-day learning adventure, beginning with foundational concepts and gradually advancing to more complex ideas. Each topic is presented in a straightforward manner, supplemented with illustrative code examples to enhance your understanding.
Question 1:
What is implicit type coercion in JavaScript, and can you illustrate it with an example?
Implicit type coercion in JavaScript occurs when the language automatically converts values from one type to another during operations. This typically happens when operators engage with operands of varying types.
For instance, using the “+” operator with differing types:
JavaScript Example:
let x = 10;
let y = '15';
console.log(x + y); // Output: "1015"
React Example:
import React from 'react';
import './App.css';
const App: React.FC = () => {
const a = 10;
const b = '15';
const result = a + b;
return (
<div className="App">
Implicit {result}</div>
);
}
export default App;
Output — 1015
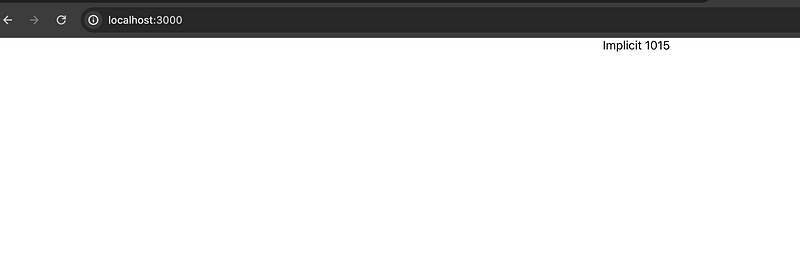
Exploring More Implicit Coercion
Here’s another example using the “+” operator:
let x = 5;
let y = '10';
console.log(x + y); // Output: "510"
React Example:
import React from 'react';
import './App.css';
const App: React.FC = () => {
const a = 10;
const b = '15';
const result = a + b;
let x = 5;
let y = '10';
console.log(x + y);
return (
<div className="App">
Implicit {result}
<br/>
Another + Example {x + y}
</div>
);
}
export default App;
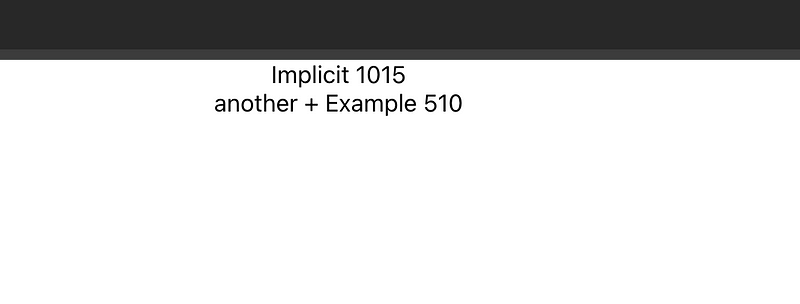
Conclusion on the “+” Operator
In the previous example with the “+” operator, even though x is a number and y is a string, JavaScript coerces x into a string, leading to the concatenated result of "510".
Let’s consider other operators:
- Subtraction:
let x = '10';
let y = 5;
console.log(x - y); // Output: 5
JavaScript converts the string '10' into a number and performs the subtraction, resulting in 5.
- Multiplication:
let x = '5';
let y = '2';
console.log(x * y); // Output: 10
Both x and y are strings, but JavaScript converts them to numbers, yielding 10.
- Division:
let x = '10';
let y = 3;
console.log(x % y); // Output: 1
The modulus operator calculates the remainder after dividing x by y, converting '10' to a number.
- Comparison Operators:
console.log(5 == '5'); // Output: true
console.log(5 != '5'); // Output: false
JavaScript coerces the string '5' into a number when using loose equality (==).
Understanding implicit type coercion is vital for avoiding unexpected behavior in your JavaScript code. Being aware of how data types are handled during operations enables you to write more robust and reliable code.
If you find this content helpful, please consider subscribing and supporting me on GitHub!
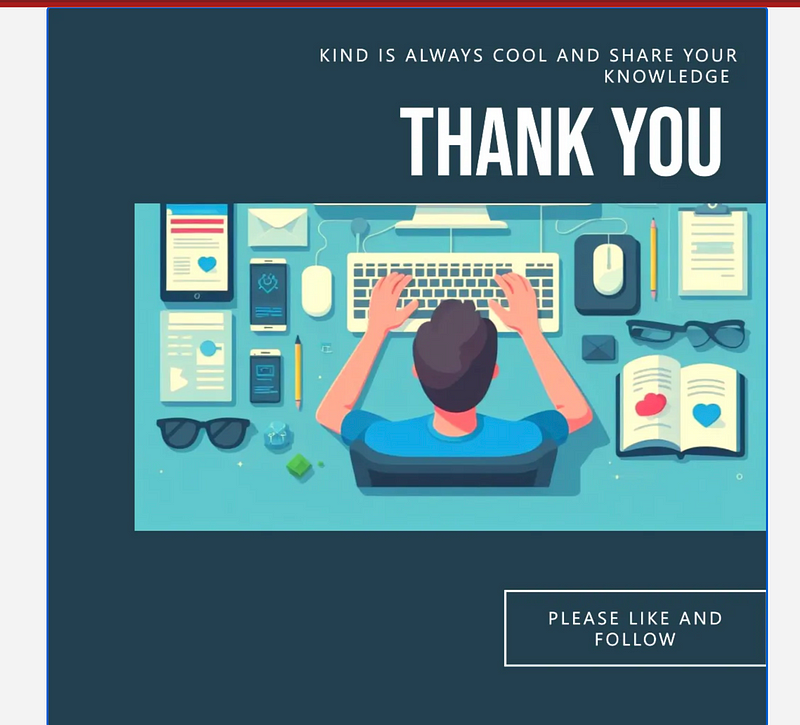
Additional Learning Resources
The first video, "JavaScript Mastery Complete Course | JavaScript Tutorial For Beginner to Advanced," provides a deep dive into JavaScript, guiding you from basic to advanced concepts.
The second video, "Learn JavaScript on the Now Platform: Lesson 1 - Getting Started," serves as a perfect introduction for beginners eager to learn JavaScript fundamentals.
Thank you for joining this journey! Remember, sharing knowledge enriches us all.