Creating a Text-Based RPG in Python: A Comprehensive Guide
Written on
Chapter 1: Introduction to Python RPG Development
In the span of 15 days, I developed an RPG game in Python. If you're eager to bypass the tutorial and dive directly into the code, you can find the project on GitHub! By the conclusion of this series, you'll be capable of crafting a text-based RPG, akin to the GIF displayed below.
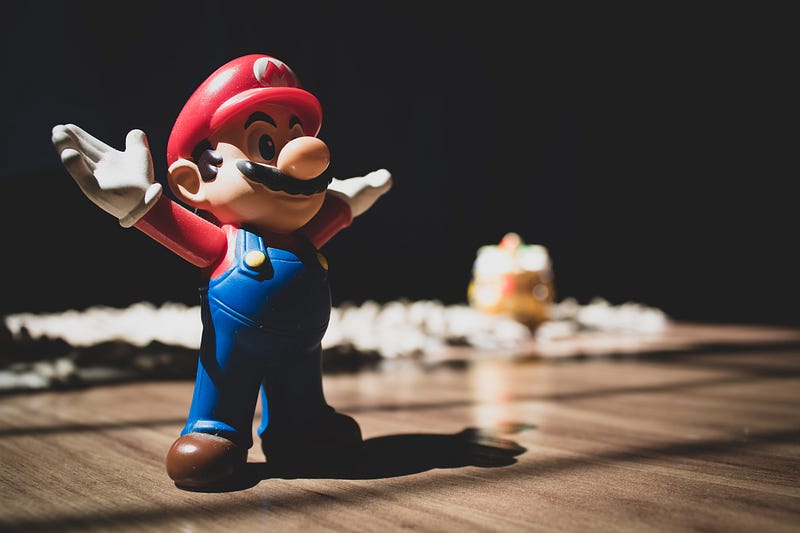
If you have any suggestions for enhancing the code or other feedback, feel free to reach out. Enjoy the article!
Chapter 2: Project Overview
In the upcoming sections, we will examine the following components: - Hero Class (including its Getters and Setters) - Enemy Class (including its Getters and Setters)
Section 2.1: The Hero Class
Hero Class Attributes: The Hero class comprises seven distinct attributes: - Health = Hhealth - Attack = Hattack - Luck = Hluck - Ranged = Hranged - Defense = Hdefence - Magic = Hmagic - Name = Hname
We will utilize these attributes to define setters and getters that allow us to access and modify the Hero's properties. For instance, if the Hero sustains damage, we can modify the "Hhealth" attribute accordingly, or increase "Hhealth" when the Hero levels up.
Getters and Setters in Python: Getters and setters help enforce validation rules when retrieving or updating values. Here's how to define a getter in Python:
def getHealth(self):
return self.health
This method, named "getHealth," will be invoked whenever we need to access the Hero's health.
To create a setter, you can use the following format:
def setHealth(self, newHealth):
self.health = newHealth
Here's an illustrative example from GeeksforGeeks that clarifies the concept of setters and getters:
class Geek:
def __init__(self, age=0):
self._age = age # getter methoddef get_age(self):
return self._age # setter methoddef set_age(self, x):
self._age = x
raj = Geek() # setting the age using setter raj.set_age(21) # retrieving age using getter print(raj.get_age()) # Output: 21 print(raj._age) # Output: 21
Hero Class Code: (Insert relevant code snippets here)
Section 2.2: The Enemy Class
Enemy Class Overview: Much like the Hero class, the Enemy class will also employ setters and getters. However, we will introduce a subclass: "The Boss."
To achieve this, we will inherit from the Enemy class as the parent class while defining the Boss class as the child class:
class Boss(Enemy):
def __init__(self, Ehealth, Eattack, Especial, Echance, Ename, EsuperMove):
super().__init__(Ehealth, Eattack, Especial, Echance, Ename)
self.superMove = EsuperMove
The first video titled "Python Text RPG (Part 1) - How to Install Python & Sublime Text" covers the installation process necessary for setting up your development environment.
The second video, "Python Text-Based RPG Tutorial Part 1 - Starting the Game," dives into the initial steps of creating your text-based RPG game.