Master the Art of C++ Programming: A Comprehensive Guide
Written on
Chapter 1: Introduction to C++
Are you prepared to begin an exhilarating journey into the realm of C++ programming? C++ stands as one of the most robust and adaptable programming languages available today. With its exceptional speed and extensive feature set, it is an ideal choice for those aiming to develop high-performance applications or systems.
But how do you initiate this journey?
The path to mastering C++ may appear overwhelming at first, particularly for newcomers. However, with the right tools and a commitment to learning, you'll soon find yourself proficient in writing C++ code.
To start, let’s cover the essentials. C++ is built on key concepts such as variables, data types, control structures, and functions. Mastering these foundational elements is crucial to your success in programming with C++.
Don't worry!
Grasping the fundamentals of C++ is more manageable than you may think. Begin by familiarizing yourself with basic data types like int, char, and double. Once you’re comfortable with these, you can progress to control structures, including if-else statements and loops. These constructs will empower you to dictate the flow of your program, allowing it to function as you intend.
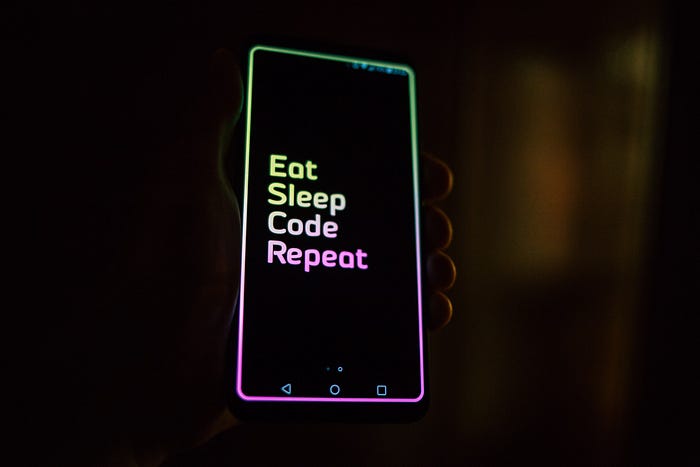
Variables in C++
In C++, variables serve as the backbone for storing and manipulating data within a program. Each variable has a specific type, such as int for integers, float for decimal numbers, or char for characters, and is referenced by a unique name. The syntax for declaring a variable in C++ is straightforward:
type variable_name;
For instance, to declare an integer variable named "age", you would write:
int age;
Once declared, you can assign a value using the assignment operator =:
age = 25;
Alternatively, you can assign a value upon declaration:
int age = 25;
Data Types in C++
In C++, data types define the kind and size of a variable. They specify what kind of data can be stored and how it is represented in memory. The primary data types include:
- int (integer): whole numbers like -1, 0, or 100.
- float: decimal numbers like 3.14 or -0.01.
- double: high-precision decimal numbers.
- char: single characters or symbols, e.g., 'a' or '$'.
- bool: a true or false value.
C++ also accommodates user-defined data types using typedef and struct or class keywords:
typedef int MyInt;
MyInt a = 10;
Or:
struct MyStruct {
int x;
int y;
};
MyStruct myStruct;
Control Structures in C++
Control structures are essential for directing the execution flow of a program in C++. They enable you to specify the order of statement execution based on conditions or events. The main types include:
- Conditional Statements: These determine which code block executes based on a condition's truth. The most common is the if statement, which runs a block of code if the condition is true. The if-else statement allows for alternative code execution based on condition evaluation.
- Looping Statements: These execute a block of code multiple times depending on a condition. Common examples include the for, while, and do-while loops.
- Jump Statements: These transfer control to different parts of the program. Examples include break, continue, and return.
Functions in C++
Functions are the core components of any C++ program. They help organize your code into manageable sections, enhancing reusability and maintainability. Understanding functions will enable you to write cleaner, more efficient code.
A function has the following characteristics:
- A name used to call the function.
- Input parameters (or arguments) that pass data into the function.
- A return value that can pass data back to the caller.
- A body that contains the code to be executed.
The basic syntax for a function is:
return_type function_name(parameter_list) {
// function body
}
For example, a function named "add" that sums two integers can be defined as:
int add(int a, int b) {
return a + b;
}
You can call functions from anywhere in your program:
int result = add(3, 4); // result will be 7
C++ also supports Function Overloading and Function Templates for added flexibility.
Arrays and Strings in C++
Arrays and strings are essential tools for managing collections of data in C++. Learning to manipulate these structures is critical for mastering C++.
Arrays consist of a collection of variables of the same type, indexed for access. For instance, to declare an array of integers, you can write:
int numbers[5];
Strings, in contrast, are sequences of characters, typically used for text. C++ provides a built-in string class for this purpose:
string name = "John";
Both arrays and strings are vital in C++ programming, and understanding their operations is crucial for developing efficient code.
Advancing Your C++ Skills
Once you grasp the basics, you can explore advanced topics like pointers, classes, and templates. These powerful features enhance your programming capabilities.
Pointers hold the memory address of another variable, enabling direct memory manipulation:
int x = 10;
int* p = &x; // p points to x
Classes allow you to define custom data types with methods and properties, promoting code organization:
class MyClass {
public:
int x;
void doSomething();
};
Templates enable the creation of generic classes and functions that work with various data types:
template<typename T>
T max(T a, T b) {
return a > b ? a : b;
}
The best way to learn C++ is through practice. The more you code and solve problems, the more skilled you will become.
C++ can be vast and complex; mastering it varies based on your background and dedication. While some programs may contain just a few lines of code, others can span millions.
Ultimately, it's not about the volume of code or time spent, but rather understanding the language's principles and applying them effectively.
So, what are you waiting for? Tap into the power of C++ and start creating impressive applications today! With the right resources and a bit of determination, you will be a C++ expert in no time.
Unlock the Power of C++ with this engaging video that introduces you to essential concepts and techniques for mastering this versatile language.
Level up your coding skills today! This short video highlights key strategies for enhancing your proficiency in C++ programming.
"Programming is not just about learning a language, it's about understanding how to think logically and solve problems. It's a discipline that teaches us how to make machines do what we want."